(Activity time: about 60 minutes)
(Download the Day One Files Here)
Intro
Hello, and welcome to the Dad and Daughter Quarantine Game Jam Tutorial!
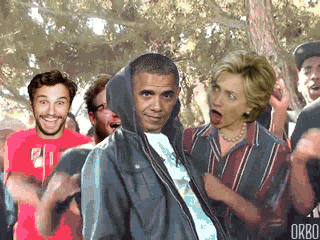
This seven-ish day tutorial will take you, the quarantined dad who knows just a little bit about programming and the quarantined daughter who wants to make games, from zero experience to one cute little game.
Obviously moms and sons and other combinations of people can do this jam. You probably don’t even need to be stuck in the house. But I write what I know.
We’re going to make a game called Mia’s Daring Escape. This is what it looks like:
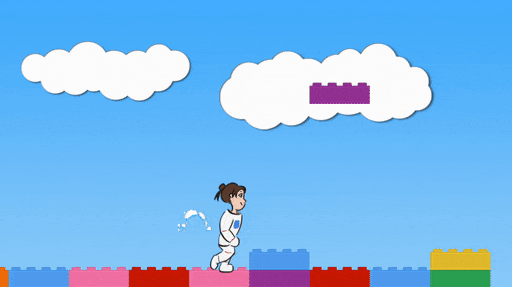
Mia is an astronaut exploring a weird toy planet when she gets caught in a hail of falling plastic bricks. Help her race back to her rocket ship before she gets bonked on the head.
This tutorial will have sections for dad and sections for kid.
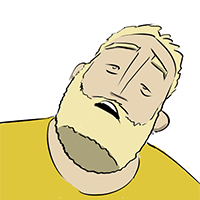
I’m providing you with a purpose built framework to make this easy, but you’re going to be learning some real javascript code.
If you and your daughter make more games, and you get some simple web space, you can put your games online and almost anyone will be able to play them from a browser.
You can teach as much or as little of this yellow text to your kid as you think is appropriate.
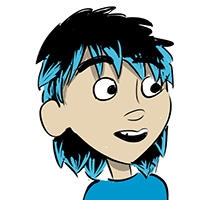
You’re going to learn how computers use numbers to run games. You’re going to learn how people like you, yes you, use carefully written sentences and lots of numbers to tell computers how to run games. It involves a lot of little magic tricks and a lot of trial and error.
Also, kid, your dad is learning. Please be kind.
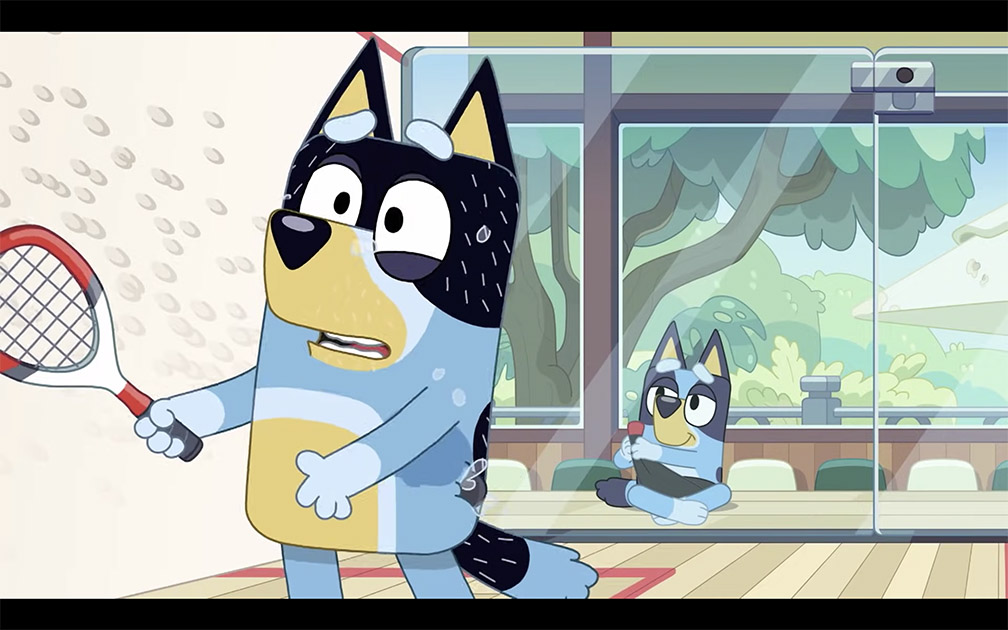
Today, Dad is going to set up the game, then Dad and Daughter are going to put Mia and some bricks on the screen together. On the way, you’ll both both learn a little about the coordinate system. Finally, Dad is going to add keyboard controls to the game, and Dad and Daughter are going to play around with changing the location of Mia and the bricks.
Let’s get started!
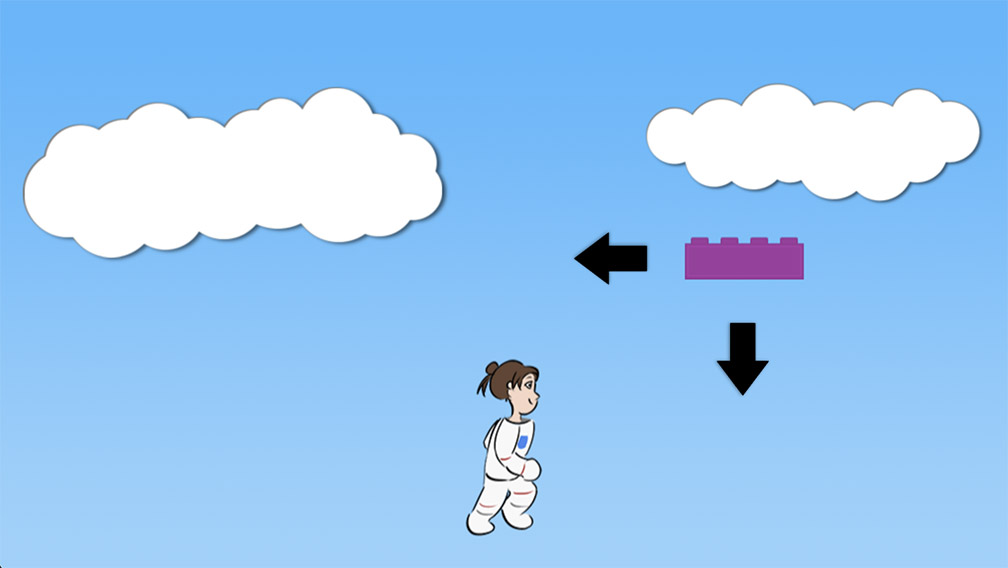
Setting Up the Game
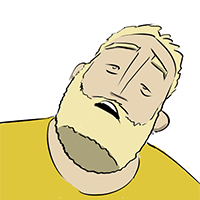
What we’re making is a browser game using the javascript programming language. It’s meant to run on a website.
But for good security reasons, you can’t just run the game directly from your laptop by opening the main file in your browser. The browser wants to see a website, not a local file.
So to make things easy, I’ve made a loader program that will run your game. You can open the loader program, drag the game’s main index.html file onto it, and the game will start running.
Here’s the Mac Loader, and here’s the Windows Loader.
You’ll also need a text editor. Notepad works pretty well. Many programmers like Sublime Text, which is nice and also free. Don’t use MS Word, because the special formatting breaks code. If you use the Mac’s TextEdit, save everything as plain text, because again, otherwise the special formatting will break the code.
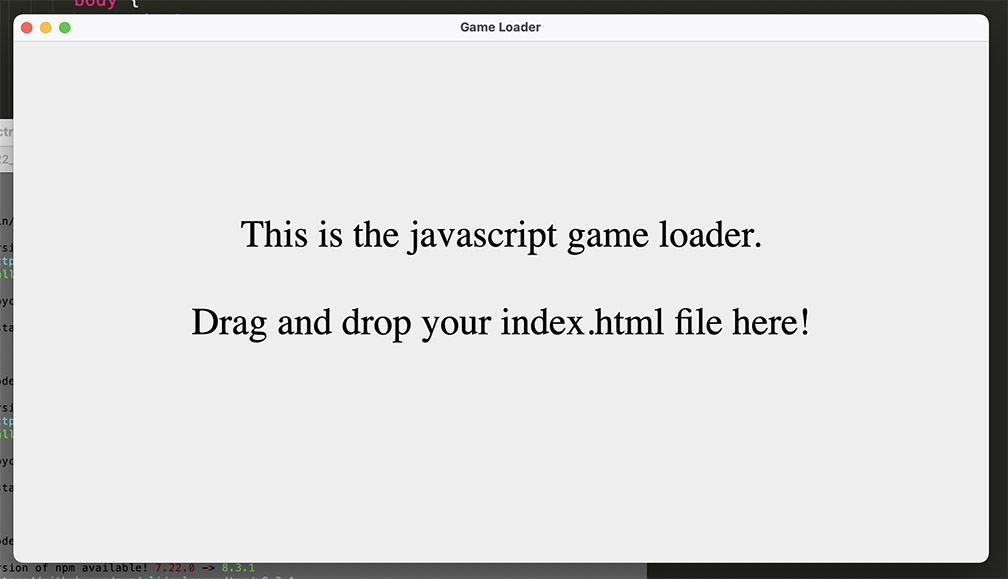
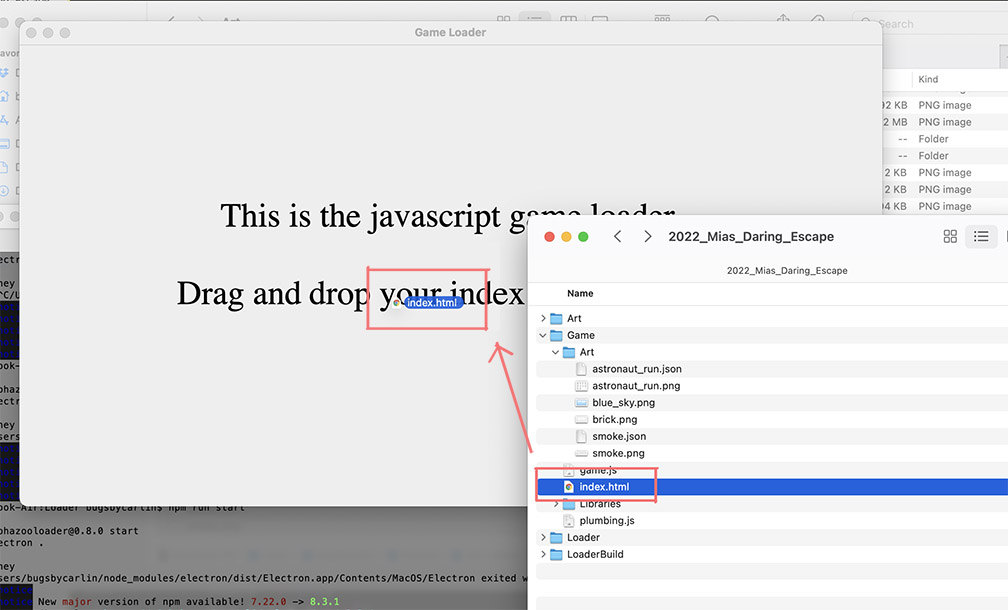
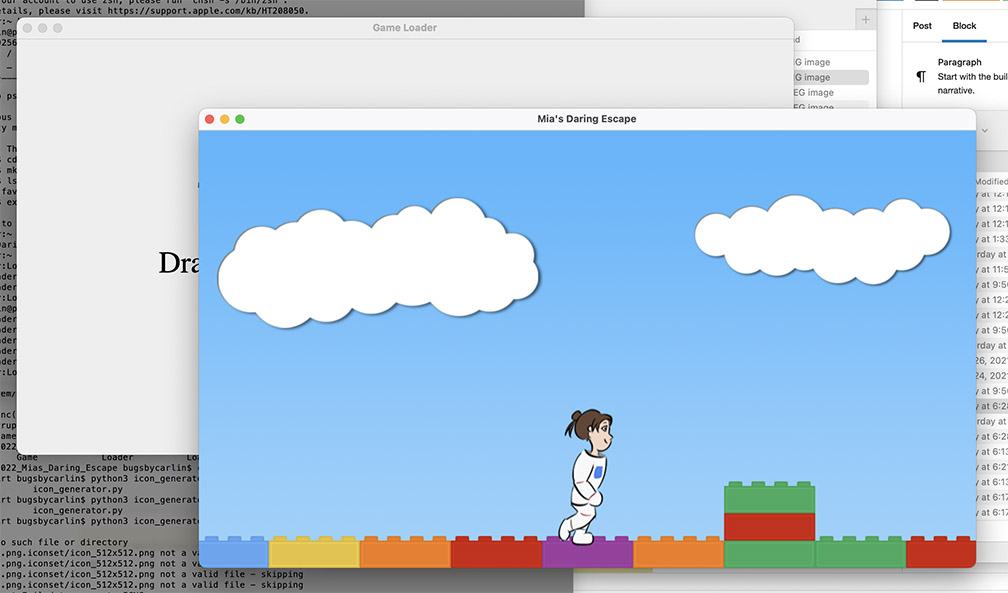
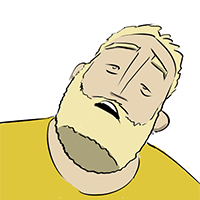
Here are the files for day one. There are art files for Mia and the brick, a source code file for you to fill in, completed source code files for reference, and a lot of helper code that you can pretty much ignore.
Download the files and put them wherever you want. Run the loader program (you may need to give it permission). Drag index.html onto the loader, and you’ll get… blue sky.
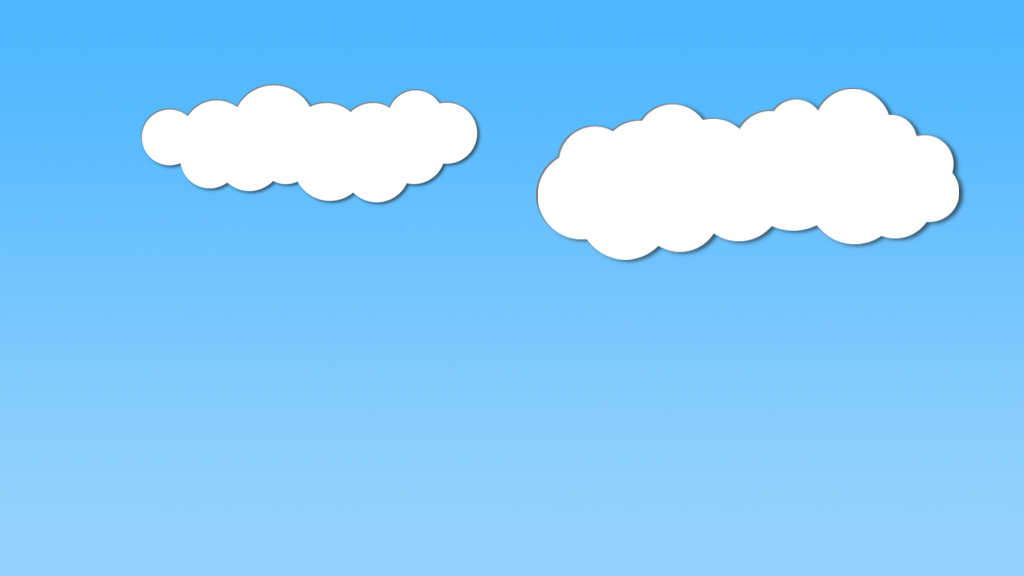
First Task: Add Mia!
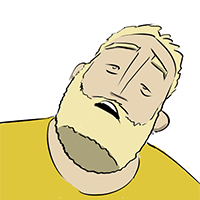
Open the file called game.js. This is where you’ll be working.
You should see something like this:
// Welcome! This is where you'll write your game code.
// Anything with two // slashes in front is a comment.
// The computer ignores comments. They're for humans
// to explain things to other humans.
function initializeGame() {
let blue_sky = makeSprite("Art/blue_sky.png");
blue_sky.position.set(0, 0);
stage.addChild(blue_sky);
}
function updateGame(diff) {
}
Detail: I’ve hidden all the code that creates the game. If you’re curious, our game is running on a framework called PixiJS, and all my extra code is hidden in the plumbing.js file. We’re going to ignore that for today.
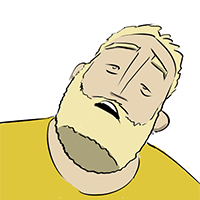
There are two functions we’re going to be working with.
A function is basically a command you can give the computer. You can put as little or as much as you want into a function, and you can name it whatever you want. It’s sort of a way of grouping thoughts together. “All of this stuff here, let’s call it MyMorningBreakfastRoutine, or OrganizeBedroom.”
To make a function, you write the word “function”, followed by a name, followed by some ( ) parentheses where you can put any extra information you want to give along with your command. Then, everything between the { } curly braces is the stuff the function actually does.
initializeGame is run one time, right when the game starts.
It has nothing between the ( ) parentheses because it doesn’t need any extra information.
In between the curly braces are three commands. First, we make a new sprite using makeSprite (another function which made which is hiding in plumbing). We give it a filename, “Art/blue_sky.png”, which is the image file for the blue sky, and it makes a sprite.
We then set the position of the blue sky to (0, 0). I’ll explain that in a minute.
Then we add the blue sky to the stage. The stage is where you put stuff on the screen. If you tell the computer to put something on the stage, it will show up on the screen. (The word stage comes from the stage of a theater play)
A sprite is a picture which you can move around. Right now, there’s just a picture of the blue sky, but we’re going to add a sprite for Mia next.
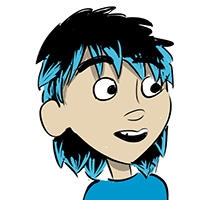
You can move sprites using numbers. For instance, you can MOVE THE SKY.
Find the line that says “blue_sky.position.set(0, 0);” and change the numbers. Try (50,50). Then, reset the game or have your Dad reset the game by pressing Command-R on a Mac or Control-R on windows. The sky should move and you should see blackness on the top left edge of the window.
Play around with a few more numbers to see where the sky goes.
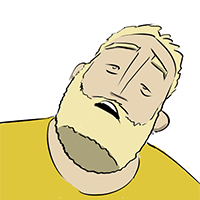
Now let’s add Mia to the game.
Add the following lines to your game code:
// Welcome! This is where you'll write your game code.
// Anything with two // slashes in front is a comment.
// The computer ignores comments. They're for humans
// to explain things to other humans.
let mia = null;
function initializeGame() {
let blue_sky = makeSprite("Art/blue_sky.png");
blue_sky.position.set(0, 0);
stage.addChild(blue_sky);
mia = makeAnimatedSprite("Art/mia_animations.json", "run");
mia.position.set(200, 200);
stage.addChild(mia);
}
function updateGame(diff) {
}
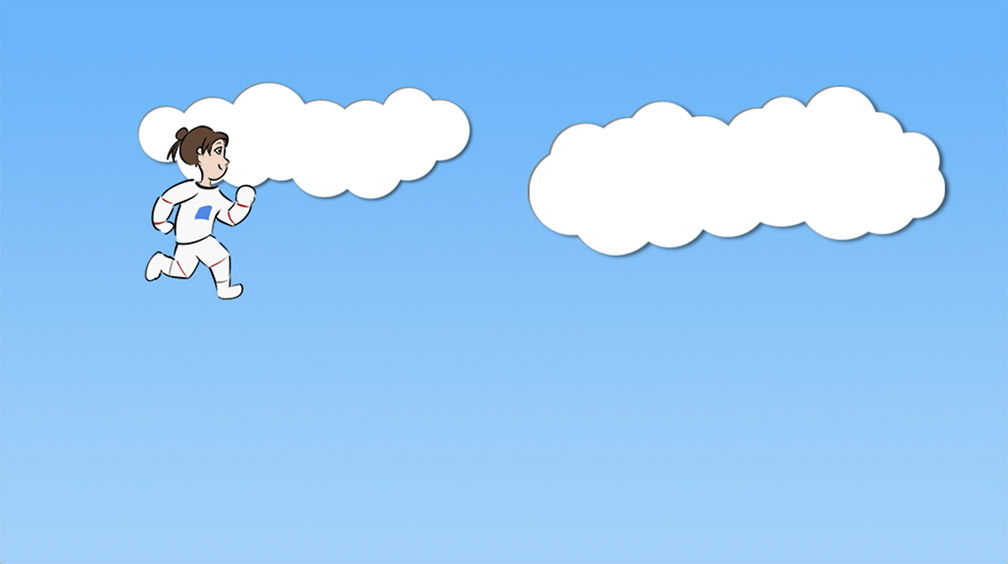
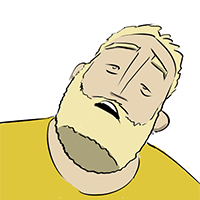
It looks the same, except we’re using a different function to make an animated sprite. This one also asks us to choose a particular animation. Mia has animations for “run”, “rise”, “fall”, and “idle”. We choose run. You can change this to see what the rising and falling parts of jump look like.
Also, near the top, we wrote “let mia = null”.
The word mia is a variable. Variables hold our stuff, whether it be numbers, text, or sprites. They’re called variable because you’re allowed to change them. For instance, you can say “cat_weight = 10;”, and then later in the program, you can say “cat_weight = 15;”, and changes the variable; now it’s 15 instead of 10.
Later, we’ll learn about “let”, and “null” and why we put this line outside the function.
There we go! Mia’s on the screen! But she’s frozen, not moving.
Let’s add two more lines.
// Welcome! This is where you'll write your game code.
// Anything with two // slashes in front is a comment.
// The computer ignores comments. They're for humans
// to explain things to other humans.
let mia = null;
function initializeGame() {
let blue_sky = makeSprite("Art/blue_sky.png");
blue_sky.position.set(0, 0);
stage.addChild(blue_sky);
mia = makeAnimatedSprite("Art/mia_animations.json", "run");
mia.position.set(200, 200);
stage.addChild(mia);
mia.animationSpeed = 0.3;
mia.play();
}
function updateGame(diff) {
}
Much better. Now Mia runs in place.
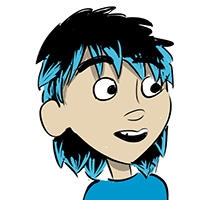
Move Mia around a little bit by using different position numbers.
Try changing the animation speed. What’s a really fast number? What’s a slow number? Is 0.1 too slow?
The numbers that change Mia’s position are part of a coordinate system. You can think of the pixels on screen like a piece of graph paper:
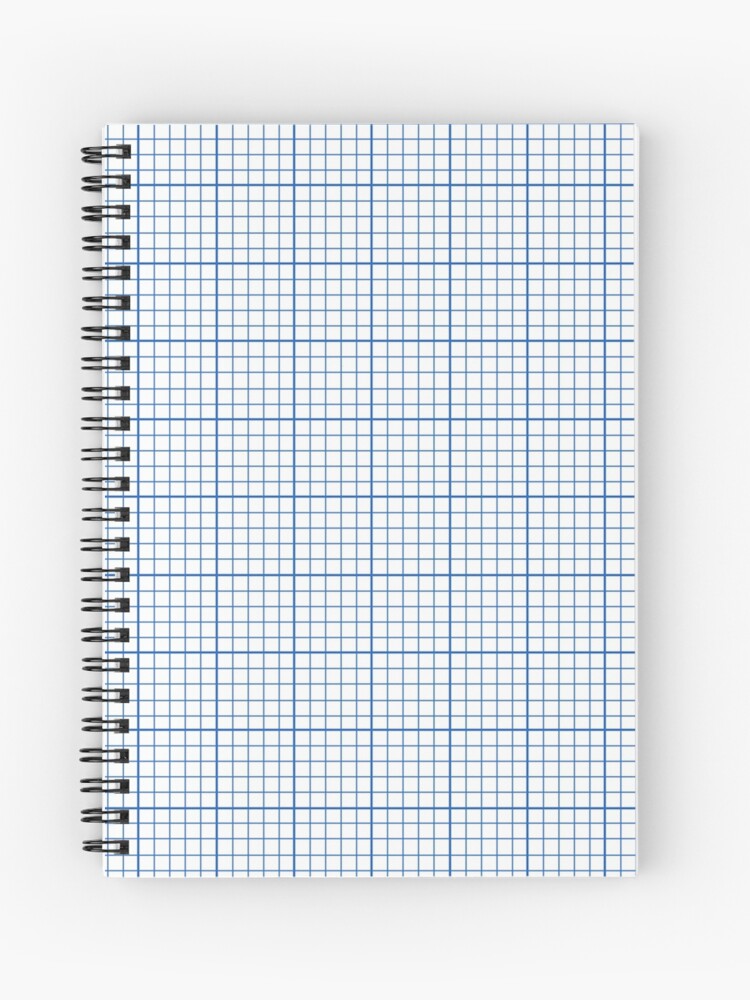
To control the location of a sprite, we use coordinates. The first one, x, controls left and right. The second one, y, controls up and down.
In Mia’s Daring Escape, the screen is 1024 pixels wide, and 576 pixels tall.
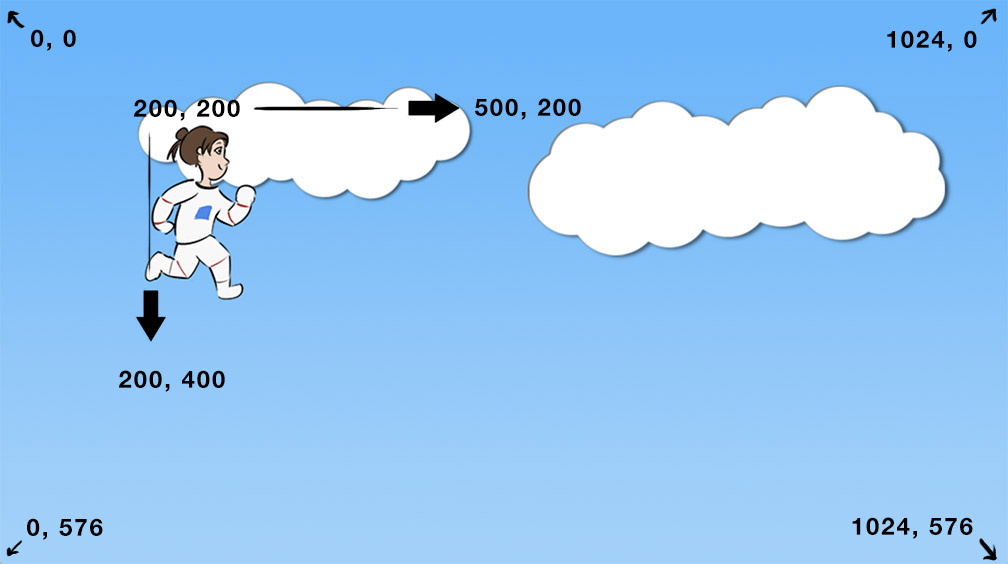
In this coordinate system, we start from the top left corner of the screen. That’s 0 on the left-right axis, and 0 on the up-down axis.
If you move to the right, the first number goes up. So, if you change Mia from 200, 200 to 500, 200, she’ll move to the right.
If you move down, the second number goes up. So, if you change Mia from 200, 200 to 200, 400, she’ll move down the screen.
Second Task: Add a brick or two!
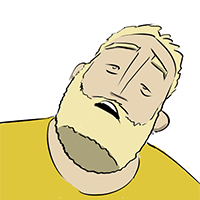
How about some bricks? Add the following code to the game.
// Welcome! This is where you'll write your game code.
// Anything with two // slashes in front is a comment.
// The computer ignores comments. They're for humans
// to explain things to other humans.
let mia = null;
let brick_1 = null;
let brick_2 = null;
function initializeGame() {
let blue_sky = makeSprite("Art/blue_sky.png");
blue_sky.position.set(0, 0);
stage.addChild(blue_sky);
mia = makeAnimatedSprite("Art/mia_animations.json", "run");
mia.position.set(200, 200);
stage.addChild(mia);
mia.animationSpeed = 0.3;
mia.play();
brick_1 = makeSprite("Art/brick.png");
brick_1.position.set(500, 200);
brick_1.tint = color(1,0,0);
stage.addChild(brick_1);
brick_2 = makeSprite("Art/brick.png");
brick_2.position.set(200, 400);
brick_2.tint = color(0,0,1);
stage.addChild(brick_2);
}
function updateGame(diff) {
}
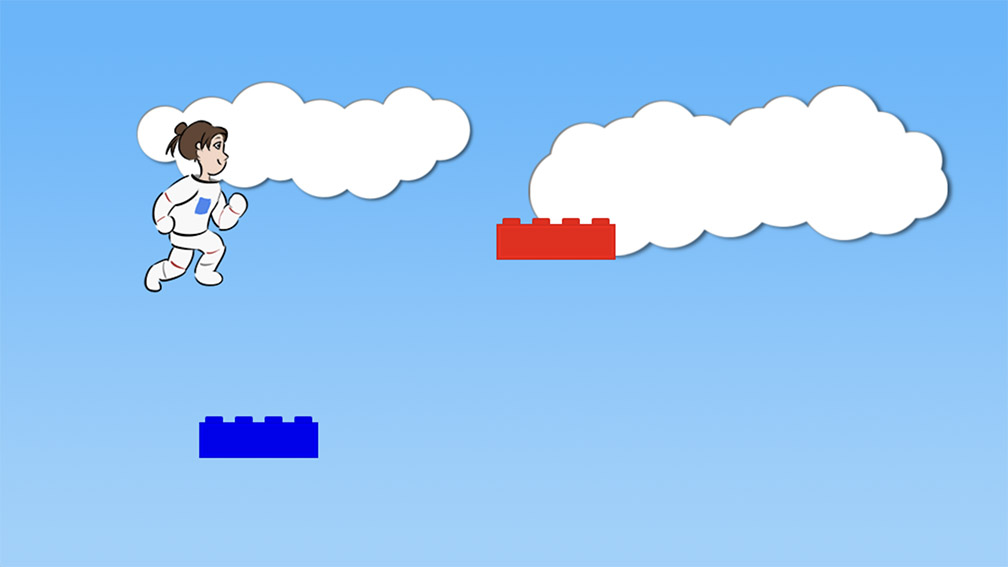
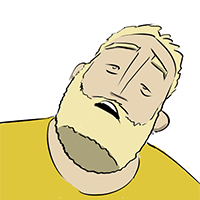
Pretty much the same stuff as before. We make two more sprites and put them on the screen. We name them brick_1 and brick_2 so we (and the computer) can tell them apart.
If you look at the actual picture we’re using, the brick is white.
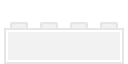
In code, we’re tinting the bricks using a color.
brick_1.tint = color(1,0,0);
color is another function. It takes three values (red, green, and blue) and makes a color number that the computer can understand.
The values range from 0 to 1. So for the first value, 0 is no red, 1 is all red, etc.
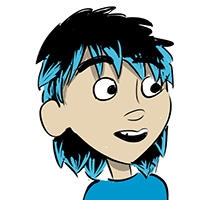
Mess around with the numbers in the color function. Can you make a green brick?
What about if we tint Mia? Try writing
mia.tint = color(0,1,0);
Alien Mia!
Last task: Make Mia move!
The last thing we’ll do today is use the keyboard to move Mia around.
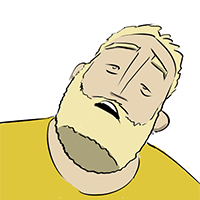
You’re probably tired, so I’m not going to explain the next part in too much detail.
<— see? You’re tired. I can see it.
Add the following to the updateGame function:
function updateGame(diff) {
// If the right key got pushed, move Mia to the right
if (key_down["ArrowRight"]) {
mia.x = mia.x + 5;
}
// If the left key got pushed, move Mia to the left
if (key_down["ArrowLeft"]) {
mia.x = mia.x - 5;
}
// If the down key got pushed, move Mia down
if (key_down["ArrowDown"]) {
mia.y = mia.y + 5;
}
// If the up key got pushed, move Mia up
if (key_down["ArrowUp"]) {
mia.y = mia.y - 5;
}
}
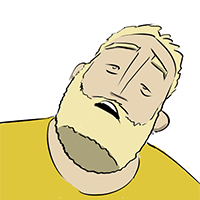
Your game is drawing and updating about 60 times a second. Every time it draws and updates, it calls the updateGame function. Anything you put in here will happen about 60 times a second.
So we’re putting keyboard actions in that bad boy. There’s code in plumbing which writes all the keyboard information into a dictionary called key_down. Basically key_down is something that can tell you if various keys have been pressed.
Don’t stress too much about the structure of this code. What it’s doing is “if the right arrow has been pressed, move Mia on the x axis by 5 pixels”, etc.
By the way, the reason we put “let mia = null” outside the initializeGame function is so we could use the “mia” variable in updateGame too. “blue_sky” lives only inside the updateGame function. But “mia” is available to use everywhere.
And there we go! Mia is moving.
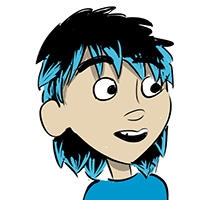
The program “listens” to your keyboard to see if you’ve pressed any keys. The code we just wrote tells the computer what to do if the keys get pressed.
We use the coordinate system to move Mia around. x is left and right, and y is up and down.
When the player presses the right arrow key, we add 5 to x, so Mia moves 5 pixels to the right.
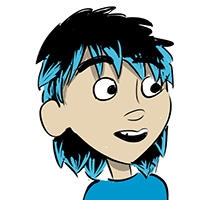
Try changing the number of pixels! If you move Mia by 10 pixels, what does it look like?
What if you use different numbers for each key?
Can you turn off the up/down movement by deleting some code?
Big challenge: can you change the code to move one of the bricks instead?
Whew. That’s a lot for day one. We’ll see you again tomorrow, when we give Mia a floor of bricks where she can run and jump!
Detail: Mia’s sprite is a tracing I made of this Fantasy Knight from itch.io. Go check it out!
Hello,
First of all, congratulations on the series.
I’ve found it on reddit today and I think this is really interesting for different kinds of publics, both newcomers and somewhat experienced developers that want to get started on game development. Given that I believe this would also benefit non-English speakers. Would you be open to translations to the tutorials and maybe adding multi-language support? Not sure how easily that could be done in the blog.
Please reach out by email if you think that idea is interesting. I’m Brazilian and I’d be interested in translating this series to Brazilian Portuguese.
Best regards,
Absolutely, will do so!